CS 106 Winter 2016
Lab 01: Arrays and Strings
Summary
This lab will allow you to practice with manipulation of arrays and strings, and the relationship between the two.
Question 1 Singles
In this question you will practice writing a few short
functions that work with strings and arrays. You'll put
your functions together into a single sketch, but the goal
is not to make that sketch actually do anything—it
will simply hold a few self-contained functions. You are
encouraged to add additional code (e.g., in a setup()
function) to test whether your functions work as expected.
With that in mind, create a folder called L01
.
Inside that folder, create a new, empty sketch called
Singles
. Inside that sketch, write the following
functions:
- Write a function
mcFace()
that takes aString
as input and returns a newString
in which the input has been used as the basis to make a phrase like "Boaty McBoatFace". For example,mcFace( "Dirk" )
should return the string "Dirky McDirkFace". Generally, any string "XXX" you pass in takes the place of "Boat", yielding "XXXy McXXXFace". - Write a function
explode()
that takes aString
as input and returns an array of characters (i.e.,char[]
) consisting of the characters in the order they originally appear in the string. For example,explode( "Dirk" )
should return the array{'D', 'i', 'r', 'k'}
. - Write a function
assemble()
that behaves likeexplode()
in reverse. It takes an array of characters as input and returns aString
consisting of all the characters in the array glued together. For example, after executingchar[] cs = { 'D', 'i', 'r', 'k' }; String s = assemble( cs );
The variables
should contain the string "Dirk".
Note that these are "pure functions". They should take inputs
as parameters (not through global variables!) and produce
outputs using a return
statement (not using
println()
or by displaying anything in the sketch
window!).
Although the Singles
sketch doesn't have to do
anything, you can still at least test that you've written legal
code by trying to run it. If Processing won't run the sketch,
presumably you've got an error somewhere in your code. If you
want to take things further, we suggest creating a
setup()
function that tests these functions by
calling them with a few different inputs (as in the examples
given above) and checking that you get back the values you expect.
If you want to practice solving self-contained problems like these, many more are available on the course web page in a set of practice programming exercises.
Question 2 Limericks
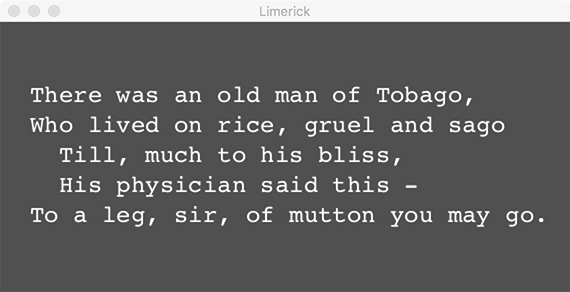
Edward Lear is perhaps best known for his poem The Owl and the Pussycat, but he also wrote volumes of (entirely wholesome, safe-for-work) limericks, and helped give that poetic form the popularity it enjoys today. You will write a sketch to display one of Lear's limericks, one line at a time.
- Pick your favourite Edward Lear limerick. For example, choose one of the limericks on this page.
- In the
L01
folder, create a new sketch called Limerick. At the top of the sketch, declare a global variable to hold an array ofString
s, with oneString
for each of the five lines of the limerick. Don't put the whole poem into a single string—use an array, with one string per line. - Add
setup()
anddraw()
functions, both initially empty. - In
setup()
, set the font, font size, and text colour you want to use to draw the limerick. Don't rely on the initial values when the sketch starts; do choose new colours and a new font. The font size must be at least 24. You should be sure to use a standard font, which you can be confident will be installed on all machines (fonts line Helvetica, Courier, Times, etc.). You can choose the font name and size in one line like this (with your font name and size substituted):textFont( createFont( "SomeFontName", 24 ) );
(See the Processing reference for more information abouttextFont()
andcreateFont()
.) -
In the
draw()
function, draw the limerick. Although we'll eventually draw it one line at a time, it's helpful to start by drawing the whole thing. Use afor
loop over the array, drawing each line of text using thetext()
function. Adjust the size of the sketch, the colours, the positioning of the lines, and the spacing between them to achieve a pleasing composition. -
Now add the line-at-a-time functionality. When the sketch starts,
the window should sit empty. When the user presses the space
bar, the sketch should display the first line and then wait.
Each press of the space bar reveals an additional line of the
limerick. Once the whole limerick is visible, pressing space should
have no further effect. Use a
keyPressed()
hook function to detect the space bar. You'll also need a global integer variable to keep track of what line you've gotten to.
The entire sketch can be written in about 40 lines of code, including the declaration of the array holding the limerick.
In addition to the core requirements above, we encourage you to experiment with optional enhancements. For some labs and assignments, we may award bonus marks for especially creative or challenging enhancements, or display them on the wall at the Stratford campus. Here are a few suggestions for enhancements. You can work on any subset of them in any order, or (better yet) invent your own new features.
- Add graphics to the sketch. You can draw a decorative border around the limerick, or add in a drawing, an illustration, etc.
- Modify the
keyPressed()
hook so that when the user presses the 'r' key, the sketch returns to its starting state, ready to walk through the limerick again. - For a harder challenge, change the line-by-line display so that the characters of the new line are also displayed one at a time, as if they were being typed on a typewriter. In addition to the variable tracking what line to draw, you'll need a second global variable tracking how many characters of the current line to draw.
- Change the sketch to walk through a sequence of limericks, switching to a new limerick each time the old one is displayed in full and the user presses space.
Submission
Please ensure that any sketches you submit compile and run. It's better to submit a sketch that runs smoothly but implements fewer required features than one that has broken code for all features. If you get partway into a feature but can't make it work, comment it out so that the sketch works correctly without it.
When you're done, please zip up the entire L01
folder into a single file called L01.zip
,
and submit that file to LEARN.
Do not submit
multiple individual files, or deviate from the sketch and folder
names given here! Feel free to re-submit
the complete zip file as many times as you like; we will mark
the final pre-deadline submission. Please consult the full
How To Submit document
for additional information.