CS 452/652 Winter 2024 - Lecture 9
Events, Clock, Idle
Feb 6, 2024 prev next
AwaitEvent
- synchronization: user tasks ↔ device
- AwaitEvent() argument and return code → design decision
- eventIds are defined by the kernel, known to the application
- return code - event-specific interpretation
- example: subtype, count, data
- micro-kernel: put as much functionality as possible in user-level tasks
- AwaitEvent() blocks calling task
- matchmaking task ↔ event
- 0, 1, N tasks waiting? store in queue (cf. SRR)?
- N tasks wait for event? complexity? unblock one or all?
- 0 tasks wait for interrupt? buffer in kernel?
- multiple same interrupts without waiting task indicate performance problem
- use task prioritity to make sure that hardware interrupts are handled promptly
- intention of AwaitEvent() is to expose device interrupts to the application
- program periodic interrupt in kernel
- can be decoupled: 1ms timer interrupt vs. 10ms clock tick
Timer Interrupt Handling
- save running task state
- read GICC_IAR to learn interrupt type
- if system timer interrupt
- update Cn compare register - overflow?
- clear interrupt via CS status register
- confirm interrupt to GICC_EOIR
- unblock task(s) waiting for event
- loop: read GICC_IAR again?
- schedule next task
- resume task
- note: system timer interrupts cannot be disabled at device
Clock Server
- API:
Time(int tid) - current time (in ticks)
Delay(tid, ticks) - delay, relative to current time
DelayUntil(tid, ticks) - delay, to absolute time
- these are Send() wrappers; tid is tid of a Clock server
- Time measured in "ticks"
Server Implementaion
- AwaitEvent() might block; server pattern?
- if server calls AwaitEvent(), it cannot Receive() new requests (e.g., Time())
- delegate event blocking to special task: Notifier pattern
Clock Notifier
for (;;) {
AwaitEvent();
Send(); // send tick to clock server
}
Clock Server
for (;;) {
Receive();
process(); // manage tasks
Reply(); // if applicable
}
- needs to manage list of suspended tasks
- DelayUntil = Time + Delay, but at server
- convencience for real-time loop
Idle/Halt
K3 overview
- Note: system with 1ms clock tick is fine as well
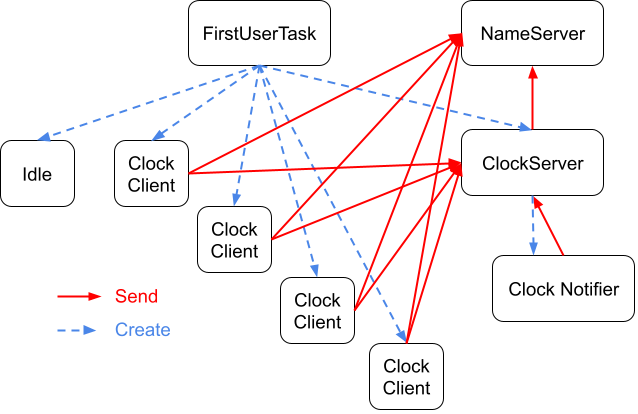